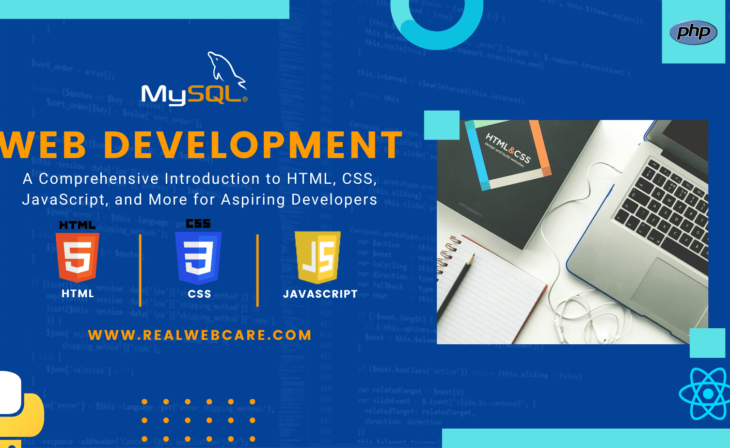
Web Development Basics: HTML, CSS, JavaScript, and More
In the vast and ever-evolving landscape of web development, two technologies stand as the cornerstones of every web developer’s skill set: HTML (Hyper Text Markup Language) and CSS (Cascading Style Sheets). Together, these foundational tools enable developers to craft the structure and aesthetics of websites, playing a pivotal role in shaping the digital world we interact with daily.
Today, I will discuss some very basic aspects of web development. By the end of this discussion, you’ll have a better understanding of what this sector entails and whether it suits you.
First, What is Web Development?
In simple terms, we all know about Facebook. Facebook is a website. Web development is the process of creating websites, like Facebook. Here, web developers are the people who work on developing websites. However, you must design a website before developing it. This means determining how the website should look and function. On the other hand, web designers are the people who work on the design of websites. Hopefully, you now understand what web development and web design are.
What Do You Need for Web Development?
Web browsers like Google Chrome, Firefox, and Safari display websites. Here, the problem is that web browsers may not be able to read or understand the designs created by designers using software like Photoshop. Therefore, web designers use a language that browsers can interpret to make their designs understandable to browsers. This language includes HTML, CSS, JavaScript, and more. Let me give you some basic ideas about these:
- HTML: HTML is like the backbone of a web page. It structures the content and tells the browser what elements to display, like headings, paragraphs, images, and links.
- CSS: CSS stands for Cascading Style Sheets. It’s used to define how the web page should look. You can set the colors, fonts, and layout of your webpage using CSS.
- JavaScript: JavaScript makes your website interactive. It allows you to add functions, animations, and user interactivity to your web pages.
You now have a basic understanding of web development and the essential tools and languages you need to know. In the next part of this discussion, I will explore these concepts in more depth and show you how to get started in web development.
Static and Dynamic Websites
We can categorize websites into mainly two types:
- Static
- Dynamic
Static Website:
The developer creates a static website exactly as it should be seen, but it cannot be changed in the future. It could be an information-rich website with your work, pictures, or videos. The developer will create it exactly as you instruct, and any changes afterward would require the same developer’s expertise. Therefore, you should carefully plan and design your static website before developing it.
Static websites can be built with just HTML and CSS knowledge, making them a good option for beginners. However, it is important to note that static websites are not ideal for websites that require frequent updates, such as e-commerce websites or blogs.
Dynamic Website:
On the other hand, you can update a dynamic website in real-time. For example, think of Facebook. You can update your status, upload pictures, delete content, and more in real-time. Dynamic websites allow users to make changes without involving the developer. They develop the site so that users can update it, delete old unnecessary items, and add new things.
To create dynamic websites, you need more than just HTML and CSS knowledge. You’ll need server-related programming languages like PHP, and you’ll also need a database, often MySQL, to handle data.
JavaScript plays a significant role in making websites attractive and interactive. Understanding graphics work using tools like Photoshop and Illustrator is also important.
If you want to freelance using only static websites, you’ll need to be highly skilled in HTML and CSS. However, to work with dynamic websites, you must become proficient in HTML, CSS, JavaScript, PHP, and possibly MySQL. Learning the basics is essential even if you don’t master everything.
Now that you have a better understanding of static and dynamic websites and what it takes to get started in web development. In the next section, we’ll explore the tools and resources you can use to begin your web development journey.
Markup Language and Programming Language:
Markup Language
HTML and CSS are markup languages. In addition, there are several other markup languages such as XML, JSON, and YAML. With HTML and CSS, whatever code you write will produce the same result. Here, you won’t achieve anything beyond that with them. These languages won’t perform real-time calculations. For example, if you ask these two languages what the result of 5 plus 5 is, they won’t be able to give you an answer. This is why they are called markup languages. However, they are vital for creating the structure and design of web pages.
Programming Language:
On the other hand, if you ask a programming language like PHP or JavaScript what the result of 5 plus 5 is, it will instantly tell you that it’s 10. In other words, PHP and JavaScript can perform real-time calculations. This is why they are called programming languages. Moreover, with languages like PHP and JavaScript, you can update content in real-time. If you want to build dynamic websites, you’ll need these programming languages along with a database like MySQL.
Now, let’s briefly discuss HTML and CSS.
HTML and CSS Coding
HTML stands for Hyper Text Markup Language. It serves as the foundation of web development, enabling the creation of structured and semantic web pages. In contrast to traditional programming languages, HTML is a markup language that provides a set of tags and elements used to structure content on the web. As a result, mastery of HTML is fundamental for anyone aspiring to become a proficient web developer. Additionally, its fundamentals are relatively easy to grasp.
Where to Write HTML Code?
You can write HTML code in a simple text editor like Notepad.
Key HTML Terminology:
HTML Element
HTML elements are the building blocks of web pages and are enclosed within tags. For instance, <h1>
is an element that defines a top-level heading, and most HTML elements follow this opening and closing tag structure.
<h1>This is a heading</h1>
Here, <h1>
is an element, and it starts with <h1>
and ends with </h1>
. Almost all elements are written this way.
HTML Attribute
Attributes provide additional information about HTML elements and are included within the opening tag. For example, <a href="https://www.example.com">This is a link</a>
contains the href
attribute, specifying the hyperlink destination.
<a title="I'm a tooltip"></a>
Here, title is an attribute that you write inside an HTML element. You need to have a good understanding of HTML elements and attributes, meaning you should have a clear idea about them.
The HTML Document Structure:
Web developers structure HTML documents hierarchically. They can nest elements within one another, creating a tree-like structure that organizes content.
When working with HTML, you typically start by creating an HTML document. Here’s a basic structure of an HTML document:
<!DOCTYPE html>
<html>
<head>
<!--- Add the JS/CSS file here -->
</head>
<body>
<h1>Content demo heading</h1>
<p>Content details go here.</p>
</body>
</html>
Now, let’s break down the components of this document:
<!DOCTYPE html>
: This declaration lets the web browser know that the document is written in HTML5, the latest version of HTML. It sets the document type for the browser to interpret the page correctly.<html>
: The root element that encloses the entire HTML document.<head>
: This section contains metadata about the document, such as the title of the page, character encoding, and links to external resources like CSS and JavaScript files.<body>
: We place the main content of the web page within this element. It encompasses text, headings, images, links, and other elements that are visible to users.
The version of HTML you’ll use depends on the declaration. For example, the above document is for HTML5. If it were for XHTML 1.0 Transitional, it would look like this:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html>
<head>
<!--- Add the JS/CSS file here -->
</head>
<body>
<!--- Add the Contents here -->
</body>
</html>
This is a transitional document, which means it allows the use of deprecated elements (elements that are no longer valid in HTML), like <font>
. If you want to use strict HTML, you would use "xhtml1-strict.dtd"
instead of "xhtml1-transitional.dtd"
.
Now, let’s look at some examples of HTML elements for a better understanding:
Common HTML Elements:
Headings
<!DOCTYPE html>
<html>
<head>
<!--- Add the JS/CSS file here -->
</head>
<body>
<h1>This is heading 1</h1>
<h2>This is heading 2</h2>
<h3>This is heading 3</h3>
</body>
</html>
Paragraphs
<!DOCTYPE html>
<html>
<head>
<!--- Add the JS/CSS file here -->
</head>
<body>
<p>This is a paragraph.</p>
<p>This is another paragraph.</p>
</body>
</html>
Links
<!DOCTYPE html>
<html>
<head>
<!--- Add the JS/CSS file here -->
</head>
<body>
<a href="https://www.realwebcare.com">This is a link</a>
</body>
</html>
Images
<!DOCTYPE html>
<html>
<head>
<!--- Add the JS/CSS file here -->
</head>
<body>
<img src="https://www.realwebcare.com/rwc-uploads/2023/09/web-development-730x448.png">
</body>
</html>
The lang Attribute
<!DOCTYPE html>
<html lang="en-US">
<head>
<!--- Add the JS/CSS file here -->
</head>
<body>
<p>This is a paragraph.</p>
<p>This is another paragraph.</p>
</body>
</html>
The href Attribute
<!DOCTYPE html>
<html>
<head>
<!--- Add the JS/CSS file here -->
</head>
<body>
<a href="https://www.realwebcare.com">This is a link</a>
</body>
</html>
Size Attributes – width/height
<!DOCTYPE html>
<html>
<head>
<!--- Add the JS/CSS file here -->
</head>
<body>
<img src="https://www.realwebcare.com/rwc-uploads/2023/09/web-development-730x448.png" width="204" height="204">
</body>
</html>
The alt Attribute
<!DOCTYPE html>
<html>
<head>
<!--- Add the JS/CSS file here -->
</head>
<body>
<img src="https://www.realwebcare.com/rwc-uploads/2023/09/web-development-730x448.png" alt="realblog">
</body>
</html>
Now, let’s move on to CSS.
CSS (Cascading Style Sheets):
CSS, short for Cascading Style Sheets, complements HTML by enabling web developers to apply styles and layouts to HTML elements. It is responsible for controlling the visual presentation of web pages, including aspects like colors, fonts, spacing, and positioning. Here are three methods of applying CSS:
Inline CSS:
Involves specifying CSS styles directly within HTML elements, like this:
<h1 style="color: blue;">This is a Blue Heading</h1>
Internal CSS:
Includes CSS rules within the <style>
element in the HTML <head>
section, like this:
<!DOCTYPE html>
<html>
<head>
<style>
body {background-color: powderblue;}
h1 {color: blue;}
p {color: red;}
</style>
</head>
<body>
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
</body>
</html>
External CSS:
Links to an external CSS file from the HTML document, like this:
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
</body>
</html>
Here, you should specify the location of your CSS file in the href
attribute. For example, if your CSS file is on your computer’s desktop, you can use a path like this:
<link rel="stylesheet" href="file:///C:/Users/YOUR_NAME/Desktop/styles.css">
For a live server, you would specify the location accordingly. Try experimenting with both internal and external CSS to understand them better.
In an external CSS file (styles.css), you would define styles like this:
body {
background-color: powderblue;
}
h1 {
color: blue;
}
p {
color: red;
}
CSS Classes:
To maintain organized and reusable styles, you can apply CSS classes to HTML elements. Web developers consider this a best practice. For instance:
<!DOCTYPE html>
<html>
<head>
<style>
div.cities {
background-color: black;
color: white;
margin: 20px 0 20px 0;
padding: 20px;
}
</style>
</head>
<body>
<div class="cities">
<h2>About Realwebcare</h2>
<p>At Real Web Care, we make it our job to listen, to research, and to understand the requirements unique to your business. We deliver high-quality web solutions and website services through our motivated, creative, and qualified specialist teams.</p>
</div>
</body>
</html>
In this example, we apply a class named “cities” to a <div>
element, and we define the styles for that class in the CSS.
These foundational concepts of HTML and CSS are essential for anyone venturing into web development. By mastering them, you’ll be well on your way to creating compelling and visually appealing websites. Armed with the knowledge of HTML, CSS, and JavaScript, you’ve taken your first steps into this dynamic domain.
But this is just the beginning. Web development is a vast and ever-evolving field. As you progress, you’ll explore advanced concepts, frameworks, and libraries that empower you to create responsive, feature-rich websites and web applications. Consider diving deeper into:
Advanced Frontend Frameworks:
Explore popular frontend frameworks like React, Angular, or Vue.js to streamline your development process and create highly interactive user interfaces.
Backend Development:
Delve into backend technologies such as Node.js, Python (Django), or Ruby on Rails to build robust server-side components that power your web applications.
Database Management:
Learn about database systems like MySQL, PostgreSQL, or MongoDB to store and retrieve data efficiently.
Version Control:
Master version control systems like Git to collaborate seamlessly with other developers and keep your codebase organized.
Web Security:
Understand web security best practices to protect your applications from potential threats and vulnerabilities.
Responsive Design:
Study responsive web design techniques to ensure your creations adapt gracefully to various screen sizes and devices.
As you continue your web development journey, remember that the web is a dynamic ecosystem, constantly evolving with new technologies and trends. Stay curious, never stop learning, and consider joining online communities, forums, or coding bootcamps to connect with fellow developers and mentors.
Whether you aspire to become a frontend specialist crafting beautiful user interfaces or a backend wizard building robust server systems, web development offers a myriad of opportunities. Your journey is unique, and the skills you acquire will empower you to bring your digital visions to life.
So, go ahead, dive into the world of web development with enthusiasm and determination. Your next web project might just be the next big thing on the internet! We wish you the best of luck on your exciting journey into the realms of web development.
There are no comments yet for this post